728x90
폼 디자인
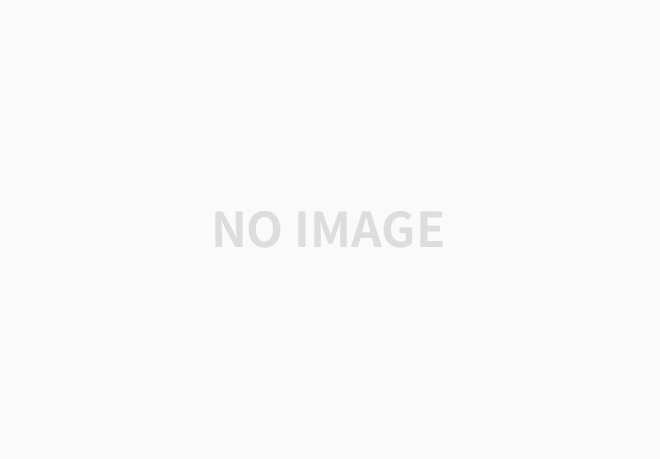
ⓐ Textbox : (Name) txtInput Readonly true
ⓑ Button : (Name) btnUpload Text file 선택
ⓒ Checkbox : (Name) chkSheetYn Checked True
ⓓ Textbox : (Name) txtSheetS Readonly True
ⓔ Button : (Name) btnConvert Text Excel to PDF 변환
소스 코드
using System;
using System.Windows.Forms;
using Excel = Microsoft.Office.Interop.Excel;
/* 1. excel 파일을 불러와
* 2. 해당 경로+ 이름에 pdf 를 붙인 이름으로
* 3. 저장한다.
*/
namespace exceltopdf
{
public partial class Form1 : Form
{
// input 파일의 경로를 저장해둘 변수 생성 후 초기화
string file_path = null;
public Form1()
{
InitializeComponent();
}
private void btnUpload_Click(object sender, EventArgs e)
{
txtInput.Clear();
file_path = null;
// openFileDialog의 시작 위치를 C:\\ 으로 설정
openFileDialog1.InitialDirectory = "C:\\";
// openFileDialog 검색 시 엑셀 파일만 검색하도록 지정해둔다.
openFileDialog1.Filter = "엑셀 파일 (*.xlsx)|*.xlsx|엑셀 파일 (*.xls)|*.xls";
// 파일 연 것 확인 진행하여 결과 값이 맞으면 시행한다.
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
// 파일선택기에서 가져온 파일 경로를 file_path 변수에 저장해둔다.
file_path = openFileDialog1.FileName;
// txtinput 에 파일 이름 보여주기 위한 코드
txtInput.Text = file_path.Split('\\')[file_path.Split('\\').Length - 1];
}
}
private void btnConvert_Click(object sender, EventArgs e)
{
if (file_path != null)
{
// pdf 파일을 저장할 경로를 지정해준다.
// 현재는 같은 경로에 같은 파일명 이름의 형태로 확장자만 변경되어 저장된다.
string export_path = file_path.Split('.')[0] + ".pdf";
Console.WriteLine(txtSheetS.Text);
Console.WriteLine(txtSheetE.Text);
// 엑셀 불러옴
Excel.Application excel = new Excel.Application();
// 엑셀 화면 띄우는 옵션. false로 띄우지 않는다.
excel.Visible = false;
// 위에서 불러온 엑셀을 연다. open(열고 싶은 파일 경로, 파일 오픈 시 ReadOnly 여부, Password 걸려있을 시 확인 메세지창 띄울건지)
Excel.Workbook workbook = excel.Workbooks.Open(file_path, true, true);
Console.WriteLine("Number of sheets: " +workbook.Worksheets.Count);
// 체크박스 체크 여부 확인 (true) -- 전체 변환 시 전체시트 excel to pdf 실행
if (chkSheetYN.Checked)
{
// 위에서 연 엑셀을 pdf로 변환하여 저장한다.
workbook.ExportAsFixedFormat(Microsoft.Office.Interop.Excel.XlFixedFormatType.xlTypePDF, export_path, Microsoft.Office.Interop.Excel.XlFixedFormatQuality.xlQualityStandard, true, true, Type.Missing, Type.Missing, true, Type.Missing);
// 실행했던 모든 파일들을 닫는다.
workbook.Close(false);
excel.Quit();
// 완료 시 판별을 위해 완료 메세지를 띄워준다.
MessageBox.Show(file_path.Split('\\')[file_path.Split('\\').Length - 1].Split('.')[0] + " 변환 완료");
}
// 체크박스 체크 여부 확인 (false) -- 텍스트박스 숫자 확인해서 해당 시트만 excel to Pdf 실행
else {
// 시트개수보다 큰 시트 번호를 입력했을 때 그냥 뱉어버림
if (Int32.Parse(txtSheetS.Text) > workbook.Worksheets.Count)
{
MessageBox.Show("1)시트 개수 2)변환하기를 원하는 시트의 번호 확인.");
}
else
{
// 입력 받은 숫자 이용하여 excel to pdf 실행
Excel.Worksheet worksheet1 = workbook.Worksheets.Item[Int32.Parse(txtSheetS.Text)];
worksheet1.ExportAsFixedFormat(Microsoft.Office.Interop.Excel.XlFixedFormatType.xlTypePDF, export_path, Microsoft.Office.Interop.Excel.XlFixedFormatQuality.xlQualityStandard, true, true, Type.Missing, Type.Missing, true, Type.Missing);
workbook.Close(false);
excel.Quit();
// 완료 시 판별을 위해 완료 메세지를 띄워준다.
MessageBox.Show(file_path.Split('\\')[file_path.Split('\\').Length - 1].Split('.')[0] +" " + " 변환 완료");
}
}
}
else
{
// file이 없을 때 버튼 클릭하면 동작x : 에러 메세지 형태로 제공한다.
MessageBox.Show("먼저 Input file을 지정해주세요.");
}
}
private void chkSheetYN_CheckedChanged(object sender, EventArgs e)
{
if (chkSheetYN.Checked)
{
// 체크가 되어있으면 시트 적어주는 textbox 초기화 + readonly
txtSheetS.Text = null;
txtSheetS.ReadOnly = true;
}
else
{
// 체크가 안되어 있으면 textbox 작성 가능하도록
txtSheetS.ReadOnly = false;
}
}
}
}
기타 기능 설정이 필요하다면
Worksheet.ExportAsFixedFormat 메서드 공식 docs
를 보고 변형 시켜보도록 하자.
결과물
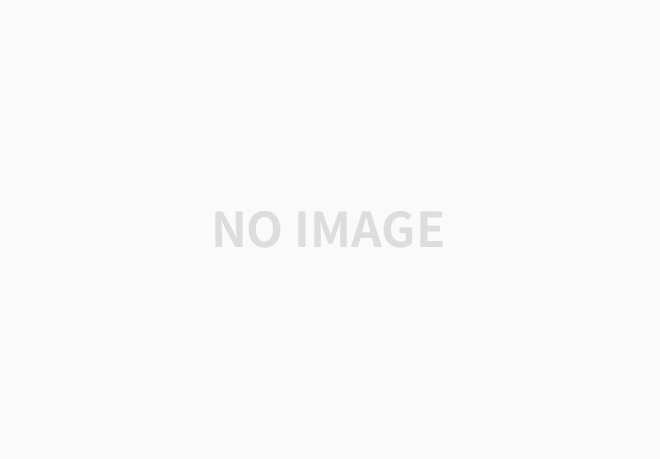
특징 및 시사점
- (아직은) 1개의 input 만 가능하다. - 배열을 사용하여 여러개 input 여러개 output 을 하도록 해야겠다
- 같은 이름으로 저장된다. 저장경로를 지정할 수 있도록 하는 것은 어떨까?
- 그냥 모든 시트를 한번에 pdf화 시키거나 지정된 시트 한개만 보낸다.. 범위 지정하여 pdf 화 하는 방법 ?
인터넷 검색해보니 쉬워보이는 방법들은 가져다쓰면 바로 될 것 처럼 모양새를 해두고는
다 유료결제를 하라고 하더라 💸💸💸💸💸
위의 시사점들을 수정하게 되면 게시글을 수정하여 보완하도록 해보겠다.
728x90